We may not have the course you’re looking for. If you enquire or give us a call on 01344203999 and speak to our training experts, we may still be able to help with your training requirements.
Training Outcomes Within Your Budget!
We ensure quality, budget-alignment, and timely delivery by our expert instructors.
The Java 8 Features have remained popular since its release in 2014. This programming language has undergone several upgrades since its conception in 1995. Out of all its previous versions, Java 8 stood out due to its plethora of exciting features. There are several Features in Java 8, some of them we discuss in the current blog.
According to the 2021 Java Technology Report by JRebel, 69% of individuals prefer Java 8 as the programming language for their primary application. The latest version of the language present today is the JAVA SE Development Kit 11.0.18. Java 8 is an old Java release, but programmers still use it widely. Let’s look at all the main features of Java 8 with examples.
Table of Contents
1) What is Java 8?
2) Java 8: Features and Examples
3) Lambda expressions
4) Functional interface
5) forEach() method
6) Default method
7) Stream Application Programming Interface (Stream API)
8) Java Date Time API
9) Nashorn
10) Conclusion
What is Java 8?
Java 8 is one of the many upgraded versions of Java. This version of Java garnered much attention owing to its multiple qualities and overall improvement as a programming language. Another interesting development was the coordination with which the libraries were updated. In the following sections, we have discussed some of the best features of Java 8.
Java 8: Features and Examples
Java 8 has various traits that can simplify multiple aspects of your work with the programming language. Increased security and ease of use are just a few of them. The following are some of the important Java 8 Features with examples:
Lambda Expressions
In the most basic terms, Lambda expressions can be defined as blocks of code that take parameters and return values. It is used to write code in a functional style. It implements a Single Abstract Method (SAM) interface using Lambda expressions and helps save code.
Syntax:
(parameters) -> expression
Or
(parameters) -> { statements; }
Here, ‘parameters’ refer to the input arguments of the Lambda expression and ‘expression’ or ‘statements’ refer to the computation performed by the Lambda expression.
Here is an example of using Lambda expressions in Java 8 to sort a list of strings in ascending order:
import java.util.*;
public class LambdaExample
{
public static void main(String[] args)
{
List
System.out.println("Before sorting: " + names);
Collections.sort(names, (String s1, String s2) -> s1.compareTo(s2));
System.out.println("After sorting: " + names);
}
}
To sort the list and pass a lambda expression as the second argument to specify the sorting order, the ‘Collections.sort()’ method is used.
The lambda expression (String s1, String s2) -> s1.compareTo(s2) takes two string arguments, ‘s1’ and ‘s2’ and returns an integer value that represents their order. To compare the two strings from the String class, the ‘compareTo()’ method returns an integer value indicating their relative order.
Output:
Before sorting: [John, Alex, Mike, Bob, Mary]
After sorting: [Alex, Bob, John, Mary, Mike]
Functional Interface
Functional interface is one of the important Java 8 Features. An interface that carries a single abstract method is called a functional interface. They are used as the basis for Lambda expressions and method references. It is also known as Single Abstract Method (SAM) Interface.
Syntax:
@FunctionalInterface
public interface MyFunctionalInterface {
public abstract void myMethod();
}
Here, ‘MyFunctionalInterface’ is a functional interface that declares a single abstract method named ‘myMethod()’. The following is an example of using a functional interface:
import java.util.function.*;
public class FunctionalInterfaceExample
{
public static void main(String[] args)
{
// Example 1: using the Predicate functional interface Predicate
System.out.println("Is 5 positive? " + isPositive.test(5)); // Output: Is 5 positive? true
System.out.println("Is -3 positive? " + isPositive.test(-3)); // Output: Is -3 positive? false
// Example 2: using the Function functional interface
Function
System.out.println("Increment 5 by one: " + incrementByOne.apply(5)); // Output: Increment 5 by one: 6
// Example 3: using the Consumer functional interface
Consumer
printString.accept("Hello, world!"); // Output: Hello, world!
}
}
In this example, three different functional interfaces are used from the java.util.function package: Predicate, Function, and Consumer. These are the functions of the three functional interfaces:
a) The Predicate functional interface takes a single input and returns a Boolean value.
b) The Function functional interface takes a single input and returns a single output.
c) The Consumer functional interface takes a single input and performs some action but returns no value.
Output:
Is 5 positive? true
Is -3 positive? false
Increment 5 by one: 6
Hello, world!
forEach() Method
The forEach() method is the default method in the iterable interface. This method receives one parameter, which is a functional interface. Collection classes can make use of the forEach loop to iterate its elements.
Syntax:
arrayList.forEach(element -> {
// code to be executed for each element
});
In the above syntax, ‘arrayList’ is the name of the ArrayList that you want to iterate over, and ‘element’ is a parameter that represents each element in the ArrayList. Here is an example of using the forEach() method:
import java.util.Arrays;
import java.util.List;
public class Example
{
public static void main(String[] args)
{
List
"Dave");
// Print out each name in the list using forEach()
names.forEach(name -> System.out.println(name));
}
}
In this example, a list of names is created, and the forEach() method is used to print out each name in the list. The forEach() method takes a lambda expression as an argument, which specifies what to do with each element in the list.
Output:
Alice
Bob
Charlie
Dave
With our Web Development Using Java Training course you can learn to build web applications using Java.
Default method
Default methods are present inside the interface and associated with a default keyword. Default methods are non-abstract methods used to define a method with the default implementation.
Syntax:
public interface MyInterface
{
// abstract method
void myMethod();
// default method
default void myDefaultMethod()
{
// default implementation
}
}
Here is an example of using default methods in Java 8. In this example, an interface 'Vehicle' declares two methods' start()' and 'stop()', which are implemented by the 'Car' class. The Vehicle interface also has a default method 'horn()', which is implemented with a 'System.out.println()' statement that prints "Beep beep!" to the console.
interface Vehicle
{
void start();
void stop();
default void horn()
{
System.out.println("Beep beep!");
}
}
class Car implements Vehicle
{
public void start()
{
System.out.println("Starting car engine...");
}
public void stop()
{
System.out.println("Stopping car engine...");
}
}
public class Main { public static void main(String[] args)
{
Car myCar = new Car();
myCar.start();
myCar.horn();
myCar.stop();
}
}
Output:
Starting car engine...
Beep beep!
Stopping car engine...
Stream Application Programming Interface (Stream API)
Functional-based operations on elements are carried out by Stream API. To understand Stream API, it is imperative to learn about Stream. One of the striking qualities of Stream is that it does not store elements; it has a functional nature and evaluates code only when required.
The syntax of Stream API has two parts. The first is creating a stream, which can be done using 'stream()' method on a collection interface, 'of()' method on a stream interface, and more. This is an example of creating a Stream using the 'stream()' method on a List interface:
List
Stream
The second part is performing operations on a Stream. You can conduct intermediate operations (filter(), map(), etc.) and terminal operations (forEach(), reduce(), etc.). Here is an example of using the ‘filter()’ method to filter out names starting with ‘S’:
Stream
Let’s try to understand this better with an example.
Following is an example of using the Java 8 Stream API to filter a list of strings based on a particular condition:
import java.util.Arrays;
import java.util.List;
public class StreamExample
{
public static void main(String[] args)
{
List
// Filter names that start with the letter 'E' List
.filter(name -> name.startsWith("E"))
.toList();
System.out.println(filteredNames);
}
}
Here, a list of strings called ‘names’ is created using the ‘Arrays.asList()’ method. Then, the ‘stream()’ method on the list is used to create a stream of elements. The ‘filter()’ method applies a filter to the stream. Finally, the filtered elements are collected into a new list using the ‘toList()’ method.
Output:
[Emily]
With our Introduction to Java EE Training course you can learn about the key concepts of Advanced Java. Sign up today!
Java Date Time API
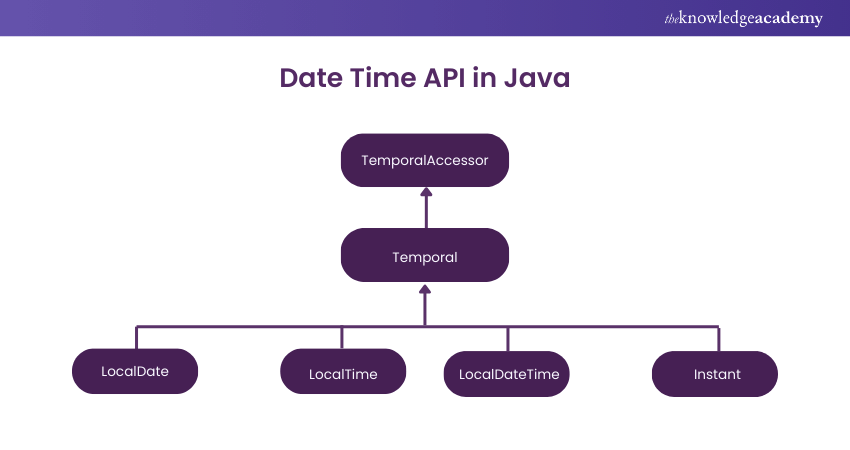
The feature of Date Time API by Java helps us represent date and time. Java 8 offers two packages - java.time and java.util. There are eight classes on the java.time package that helps you deal with date and time.
The following is an example of using Java Time API to create a date-time object using the current date and time:
import java.time.LocalDateTime;
LocalDateTime now = LocalDateTime.now();
Now let’s look at an example that deals with obtaining the current date and time and formatting it using a ‘DateTimeFormatter’.
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class JavaTimeExample
{
public static void main(String[] args)
{
LocalDateTime now = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = now.format(formatter); System.out.println("Current date and time: " + formattedDateTime);
}
}
In the above example, a ‘LocalDateTime’ object representing the current date and time is created using the ‘now()’ method. Then, a ‘DateTimeFormatter’ object with a specific pattern is created using the ‘ofPattern()’ method. Finally, the ‘LocalDateTime’ object is created using the ‘format()’ method and the ‘DateTimeFormatter’ object.
Output:
Current date and time: 2023-03-14 14:05:23
Nashorn
Nashorn is an improvised JavaScript engine. It helps directly compile code in memory and passes the bytecode to Java Virtual Machine (JVM). The command line tool to execute JavaScript code is jjs. The following is an example of using Nashorn in Java 8:
import javax.script.ScriptEngine;
import javax.script.ScriptEngineManager;
import javax.script.ScriptException;
public class NashornExample
{
public static void main(String[] args)
{
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("nashorn");
try
{
engine.eval("print('Hello, World!')");
} catch (ScriptException e)
{
e.printStackTrace();
}
}
}
In the above example, the necessary classes for using Nashorn, which are 'ScriptEngine', 'ScriptEngineManager', and 'ScriptException' are imported. Next, a new 'ScriptEngineManager' object, responsible for managing the script engines, is created.
The 'nashorn' script engine is retrieved by calling the 'getEngineByName' method on the 'ScriptEngineManager' object. Then, a simple JavaScript program is executed that prints "Hello, World!" to the console by calling the 'eval' method on
java 8 features
the 'ScriptEngine' object.
Output:
Hello, World!
Conclusion
The Java 8 features have helped Java gain traction as a programming language in various domains. More qualities of Java 8 raise its credibility. We hope this blog has helped you learn about some of the features of Java 8.
Improve your understanding of JavaScript. Sign up for our JavaScript for Beginners course now!
Frequently Asked Questions
Upcoming Programming & DevOps Resources Batches & Dates
Date
Mon 9th Dec 2024
Mon 6th Jan 2025
Mon 13th Jan 2025
Mon 20th Jan 2025
Mon 27th Jan 2025
Mon 3rd Feb 2025
Mon 10th Feb 2025
Mon 17th Feb 2025
Mon 24th Feb 2025
Mon 3rd Mar 2025
Mon 10th Mar 2025
Mon 17th Mar 2025
Mon 24th Mar 2025
Mon 7th Apr 2025
Mon 14th Apr 2025
Mon 21st Apr 2025
Mon 28th Apr 2025
Mon 5th May 2025
Mon 12th May 2025
Mon 19th May 2025
Mon 26th May 2025
Mon 2nd Jun 2025
Mon 9th Jun 2025
Mon 16th Jun 2025
Mon 23rd Jun 2025
Mon 7th Jul 2025
Mon 14th Jul 2025
Mon 21st Jul 2025
Mon 28th Jul 2025
Mon 4th Aug 2025
Mon 11th Aug 2025
Mon 18th Aug 2025
Mon 25th Aug 2025
Mon 8th Sep 2025
Mon 15th Sep 2025
Mon 22nd Sep 2025
Mon 29th Sep 2025
Mon 6th Oct 2025
Mon 13th Oct 2025
Mon 20th Oct 2025
Mon 27th Oct 2025
Mon 3rd Nov 2025
Mon 10th Nov 2025
Mon 17th Nov 2025
Mon 24th Nov 2025
Mon 1st Dec 2025
Mon 8th Dec 2025
Mon 15th Dec 2025
Mon 22nd Dec 2025